Onboard a Contractor-Only Company
Users can create contractor-only companies without W-2 employees. This company type has fewer onboarding requirements compared to those with W-2 employees. However, contractor-only companies have a payroll blocker that prevents them from processing payroll for W-2 employees.
We also enable updating a contractor-only company to a company that supports payments for contractors and payroll for W-2 employees. Note that after updating, the company must complete additional onboarding steps before they can run payroll for W-2 employees.
Contractor-Only APIs
We have not explicitly blocked APIs for contractor-only companies, but some behaviors that should be avoided. For instance, users can technically create an employee for a contractor-only company through the API. However, they should refrain from doing so since the company is contractor-only.
Although we are not explicitly blocking API endpoints, fully onboarded contractor-only companies are unable to run payroll due to the contractor-only payroll blocker. This applies even when the company has onboarded contractors.
1. Create a contractor-only company
To create a contractor-only company, specify “contractor_only”: true
within the body params of the POST /v1/partner_managed_companies
endpoint. The default case, if not specified, is “contractor_only”: false
(i.e. the company has at least one W-2 employee).
curl --request POST \
--url https://api.gusto-demo.com/v1/partner_managed_companies \
--header 'accept: application/json' \
--header 'content-type: application/json'\
--header 'Authorization: Bearer {{system_access_token}}'\
--data '{
"user": {
"last_name": "Mushnik",
"first_name": "Mister",
"email": "[email protected]"
},
"company": {
"name": "Mushnik`s Flower Shop",
"ein": 910532465,
"contractor_only": true
}
}'
const fetch = require('node-fetch');
const url = 'https://api.gusto-demo.com/v1/partner_managed_companies';
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'content-type': 'application/json',
authorization: 'Bearer <<API_TOKEN>>'
}
},
body: JSON.stringify({
user: {
first_name: 'Mister',
last_name: 'Mushnik',
email: '[email protected]'
},
company: {
name: "Mushnik\'s Flower Shop",
ein: "910532465"
}
})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
2. Get a company’s contractor_only attribute
Make a call to the GET /v1/companies
endpoint to identify if a company is contractor-only. In the response, the “contractor_only”
attribute is used to determine whether or not the company is contractor-only. It will be true
for a contractor-only company.
{
"contractor_only": true
}
3. Get a company’s onboarding requirements
You can retrieve the company’s onboarding requirements using the using the GET companies/{company_uuid}/onboarding_status
endpoint. The onboarding steps returned will be different for a contractor-only and non contractor-only company.
For a contractor-only company, the onboarding steps include add_addresses
, federal_tax_setup
, select_industry
, add_bank_info
, add_contractors
, sign_all_forms
, and verify_bank_info
.
The state_setup
, payroll_schedule
, and add_employees
steps are not included for contractor-only.
{
"uuid": "8e75cd53-9427-40a1-9f7c-4542d56d7e6e",
"onboarding_completed": false,
"onboarding_steps": [
{
"title": "Add Your Company’s Addresses",
"id": "add_addresses",
"required": true,
"completed": true,
"skippable": false,
"requirements": []
},
{
"title": "Enter Your Federal Tax Information",
"id": "federal_tax_setup",
"required": true,
"completed": true,
"skippable": false,
"requirements": []
},
{
"title": "Select Industry",
"id": "select_industry",
"required": true,
"completed": true,
"skippable": false,
"requirements": []
},
{
"title": "Add Your Bank Account",
"id": "add_bank_info",
"required": true,
"completed": true,
"skippable": false,
"requirements": []
},
{
"title": "Add Your Contractors",
"id": "add_contractors",
"required": false,
"completed": true,
"skippable": false,
"requirements": [
"add_addresses"
]
},
{
"title": "Sign Documents",
"id": "sign_all_forms",
"required": true,
"completed": true,
"skippable": false,
"requirements": [
"federal_tax_setup",
"add_bank_info"
]
},
{
"title": "Verify Your Bank Account",
"id": "verify_bank_info",
"required": true,
"completed": true,
"skippable": false,
"requirements": [
"add_bank_info"
]
}
]
}
4. Get a company’s payroll blockers
Users can retrieve a company’s payroll blockers through the GET /v1/companies/{company_uuid}/payrolls/blockers
endpoint. If a company is contractor-only, it will have the following payroll blocker:
[
{
"key": "contractor_only_company",
"message": "Company is set to contractor_only and cannot run payroll. Update the company and complete additional onboarding steps to process payroll."
}
]
This payroll blocker does not apply to companies with W-2 employees.
5. Update a Company to Support Payroll for W-2 Employees
If a contractor-only company wants to onboard and run payroll for W-2 employees, the company must first be updated using the PUT /v1/companies
endpoint
The user should specify “contractor_only”: false
in the body params.
curl --request PUT \
--url https://api.gusto-demo.com/v1/companies/company_id \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"contractor_only": false
}
const fetch = require('node-fetch');
const url = 'https://api.gusto-demo.com/v1/companies/{company_uuid}/signatories';
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'content-type': 'application/json',
authorization: 'Bearer <<COMPANY_API_TOKEN>>'
},
body: JSON.stringify({
home_address: {
street_1: '35 Fantine Street',
street_2: 'Suite 17',
city: 'Paris',
state: 'TX',
zip: '75460'
},
ssn: '254324601',
first_name: 'Jean',
last_name: 'Valjean',
email: '[email protected]"',
title: 'Business Man',
phone: '8002331000',
birthday: '1983-12-01'
})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
Updating the "contractor_only" attribute
Note that the
contractor_only
attribute can only be updated fromtrue
tofalse
, and not vice versa. In other words,contractor_only
cannot be enabled for an existing company. Also note that if you update"contractor_only": false
you must also complete thestate_setup
,payroll_schedule
, andadd_employees
onboarding steps before running payroll.
Contractor-Only Onboarding Flow
After creating a contractor-only company using the POST /v1/partner_managed_companies
endpoint, generate a company_onboarding
flow using our POST /v1/companies/{company_uuid}/flows
endpoint. The flow will have different requirements depending on whether the company is contractor-only.
curl --request POST
--url <https://api.gusto-demo.com/v1/companies/{{company_uuid}}/flows>
--header 'Authorization: Bearer {{access_token}}’'
--header 'Content-Type: application/json'
--data '
{
"flow_type": "company_onboarding"
}
const fetch = require('node-fetch');
const url = 'https://api.gusto-demo.com/v1/companies/{company_uuid}/signatories';
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'content-type': 'application/json',
authorization: 'Bearer <<COMPANY_API_TOKEN>>'
},
body: JSON.stringify({
home_address: {
street_1: '35 Fantine Street',
street_2: 'Suite 17',
city: 'Paris',
state: 'TX',
zip: '75460'
},
ssn: '254324601',
first_name: 'Jean',
last_name: 'Valjean',
email: '[email protected]"',
title: 'Business Man',
phone: '8002331000',
birthday: '1983-12-01'
})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
Non Contractor-Only Flow:
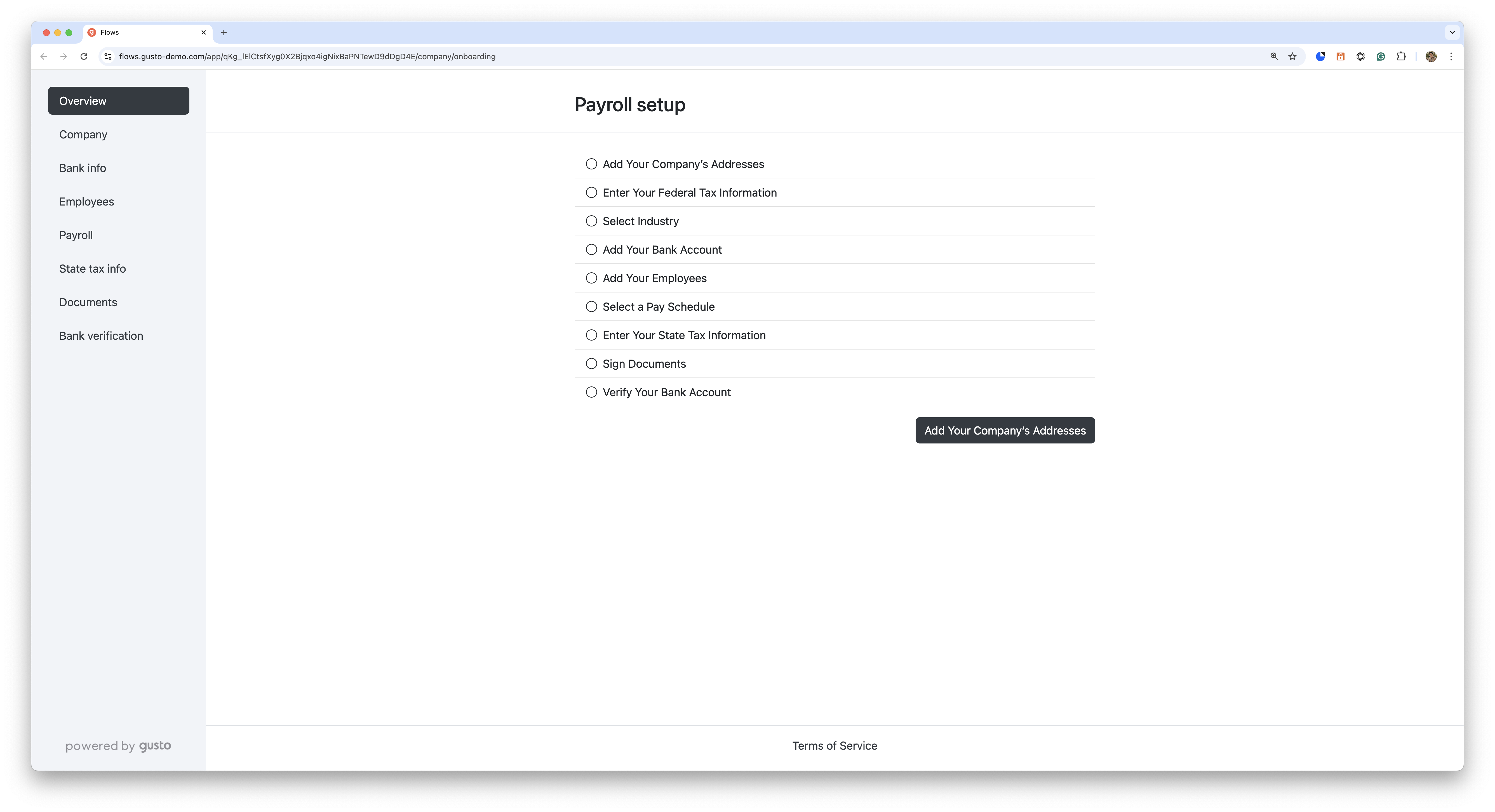
Contractor-Only Flow:
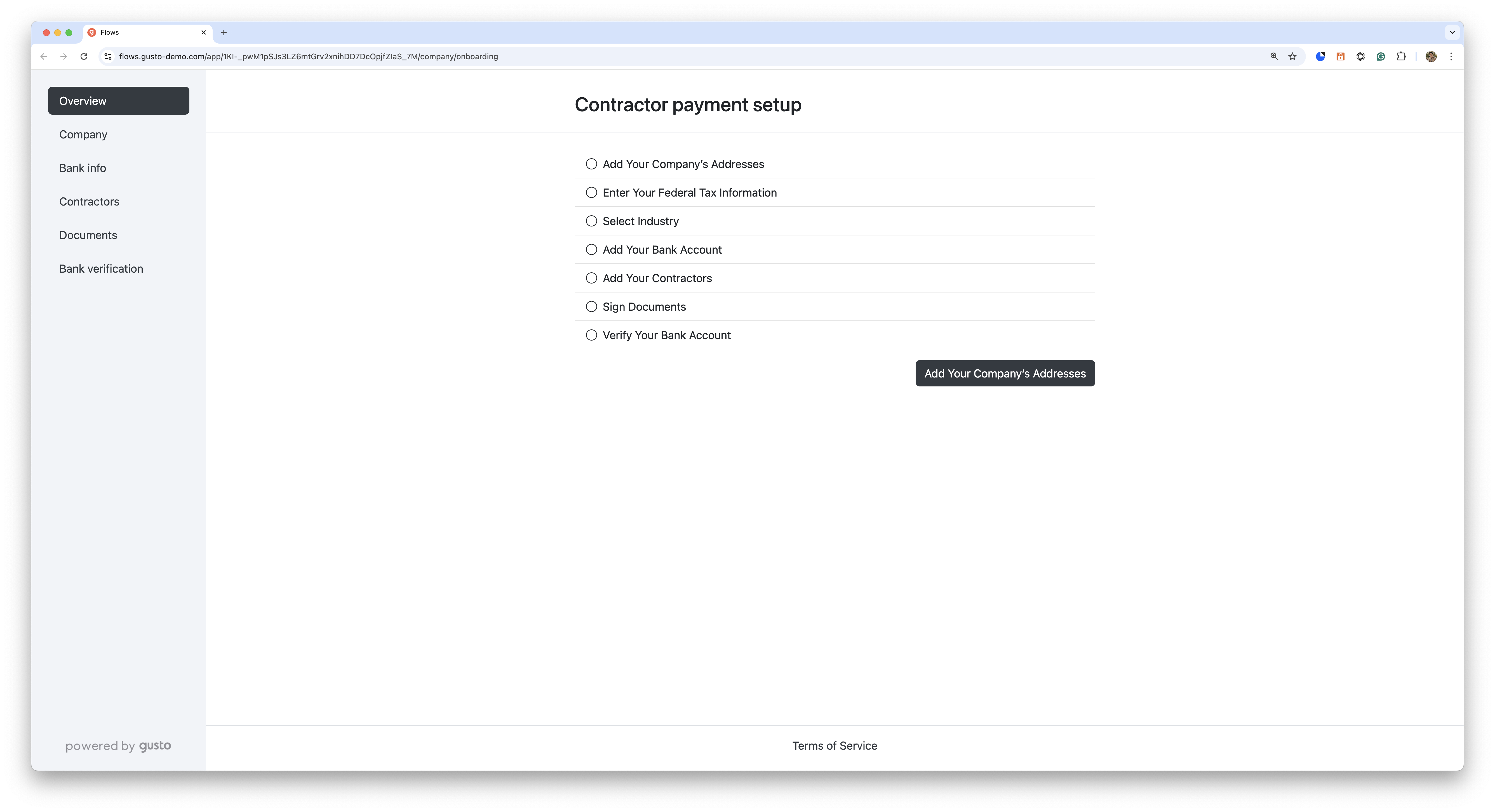
- “Add Your Employees,” “Select a Pay Schedule,” and “Enter Your State Tax Information” are not included as steps in the contractor-only onboarding flow since these steps are not applicable.
- The contractor-only onboarding flow includes the“Add Your Contractors” step that is not included in the default onboarding flow.
- We have nested the add_contractors flow within the onboarding flow.
Updated 7 months ago