Flow events
If you integrate flows with an iframe wrapper in your application, most of the work will be done for you already. Butβhow do you know when the user is done with the workflow if you need to trigger navigation or close a containing modal in your application?
Enter flow events: you can listen for a flow event signaling that the user is done with the workflow and your application can react accordingly. Flow events leverage the browser API postMessage to inform the parent application of an event in the frame This sends a message that you can receive with an event listener in Javascript.
To use flow events, you must:
- Enable flow events on your Developer Portal's Flow settings
- Add an event listener in your application to listen for 'message' events
When flow events are triggered
Flow events can be triggered automatically or manually. Refer to the Flow event trigger column in the Flow Types page to see how a flow event is triggered for a given flow. The possible values for that column are
- None: This flow is not currently supported
- Manual: The user must click a button inside the flow to trigger the event
- Automatic: The event is triggered once the user reaches the end of the flow
Automatically vs. manually
-
Automatically: The flowβs goal has been clearly completed and there is no other information to view or action for the user to take. An example is the industry selection flow: once the industry has been saved successfully, there is nothing else to be done. The flow is completed and the event is triggered automatically without additional user interaction
-
Manually: The user takes an action that signals they've finished their interaction with the flow. For flows that are more open-ended or have information that the user should consume after taking an action, flow events can be triggered by clicking a button signaling they have completed their interaction with the flow.
-
An example of this is the
add_employees
flow. Since we can't automatically determine when the user has finishedβthey may be editing many employees, adding one or multiple employees, or just viewing employee informationβthe user must inform us that they are done with their operation by clicking a button, commonly labeled Finish.
-
Structure of a flow event
Here is a raw event fired from within a flow:
{
"event": "gusto:flow-finish",
"metadata": {
"flowType": "industry_selection",
"companyUuid": "4206ae3f-5f02-4cac-b22f-3a2676449f2f"
}
}
A flow event is a JSON object. The βeventβ attribute contains the type of event fired. The βmetadataβ attribute will at minimum contain βflowTypeβ and βcompanyUuidβ, but may contain more attributes depending on the flow. If so, this will be covered in the documentation.
Currently gusto:flow-finish
and gusto:payroll-submitted
are the only flow event types, but your application should consider future expansion to these types when interpreting events.
Adding an event listener
To receive flow events when embedding flows in your application, add an event listener for βmessageβ events. Itβs also important to follow all security recommendations for verifying any eventβs origin. See the MDN postMessage documentation for more details and documentation.
window.addEventListener( 'message', (event) => {
if (event.data.event == 'gusto:flow-finish') {
// event origin verification
console.log( 'received flow event: ', event)
}
}
)
<style>
.callout-icon {
font-size: var(--emoji,1.5);
color: var(--icon-color,inherit)!important;
margin-top: 0.25em!important;
}
</style>
<blockquote class="callout callout_default" theme="π">
<h3 class="callout-heading">
<span class="callout-icon" >
<img src="https://files.readme.io/193f880-BookGuava.svg" width="23px" >
</span>
</h3>
<p>You might already have βmessageβ event listeners. Since Javascript event listeners are processed in the order that they are declared in your code, this could become an issue if, for instance, you have two listeners capable of changing the applicationβs state (such as reloading the page or changing the location).</p>
</blockquote>
Enabling flow events
Flow events are disabled by default, but you can enable them for your application by going into Flow settings in your developer portal account. Check the Enable flow events on supported flows to enable events for your application.
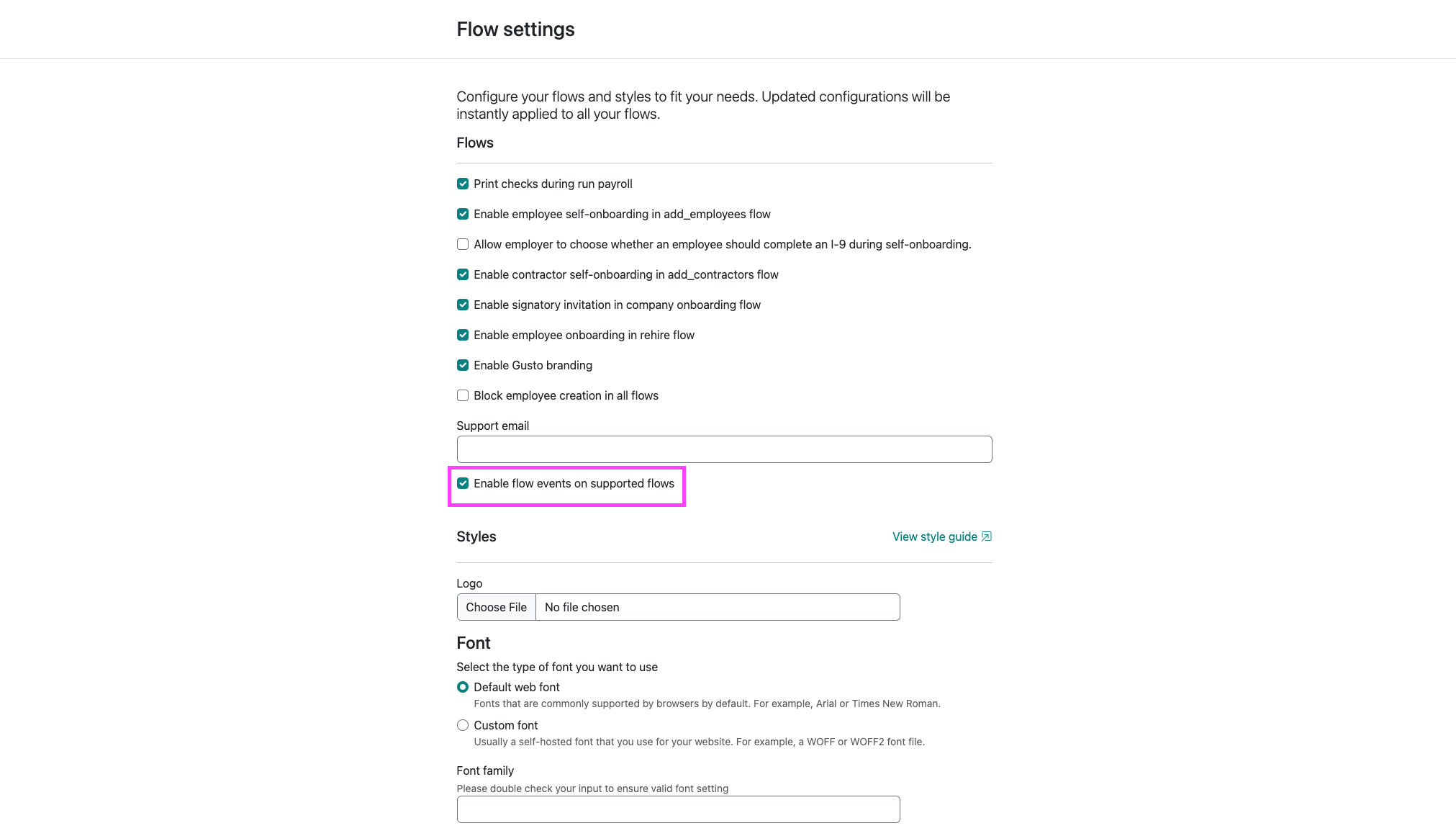
Compatibility
This functionality is ubiquitous in desktop and mobile browsers, so you can rest assured that all your customers will be covered by flow events, regardless of their computing device.
Source: Browser compatibility chart on MDN
Updated 22 days ago