Webhooks
Efficiently integrate Gusto's real-time event data into your application
Overview
A webhook is an HTTP request that delivers information about an event as it happens. We recommend that you integrate with Gusto’s webhooks infrastructure so that your application can perform subsequent business logic after it receives a webhook event.
See a list of all webhook event types that Gusto offers here.
You can only create a webhook subscription if you have one application under your organization. Having more than one application will return an error.
Event payload
It’s possible that a single API request will generate multiple webhook events. For example, when a partner-managed company is created, we may push multiple events to your application.
Here is an example of a webhook event payload:
{
"event_type": "company.provisioned",
"resource_type": "Company",
"resource_id": 7756341741171842,
"resource_uuid": "5df911a0-e8e9-412d-8aaf-a46ad193dd96",
"entity_type": "Company",
"entity_id": 7756341741171842,
"entity_uuid": "5df911a0-e8e9-412d-8aaf-a46ad193dd96",
"timestamp": 1671058841,
}
The company.provisioned
event indicates that an OAuth connection has been established between the company and the partner. Note that this event is always fired, regardless of whether your application has a subscription to the Company entity type.
For all webhook events, the resource_type
will be Company. The field entity_type
refers to the underlying API resource with the state change.
Getting started
Before setting up your first webhook subscription, please review the best practices section located at the bottom of this page.
The easiest way to get started with webhooks is by using our Developer Portal. If you would prefer to set up via the API instead, please see the Setting up via the API section below.
Setting up via the Developer Portal
To get started, sign into your Developer Portal account. Click into the Application that your webhook will belong to, and select the “Webhook subscriptions” tab. This tab will allow you to add, verify, edit, and view your webhook subscriptions.
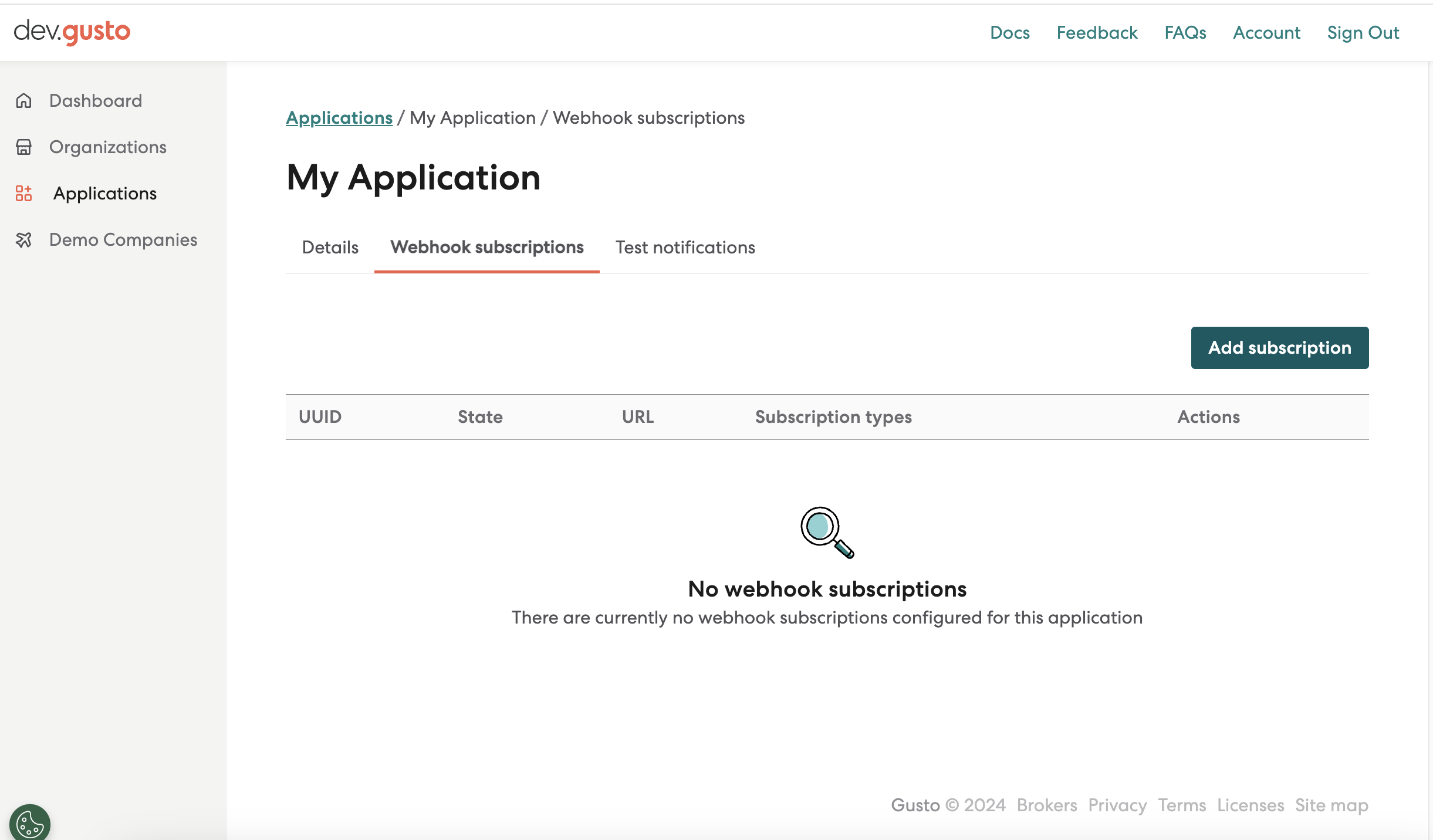
Once your verified subscription starts receiving events, you will be able to view them in your subscription’s details page, accessible via the Actions menu for a given subscription.
You can use the Event activity table to view the last n events received and filter to specific subscription types using the Search by event bar.
Please note: Your subscription must be subscribed to the subscription type of interest in order to be notified of those events. To access all events, regardless of your subscription status, you can call our Events endpoint.
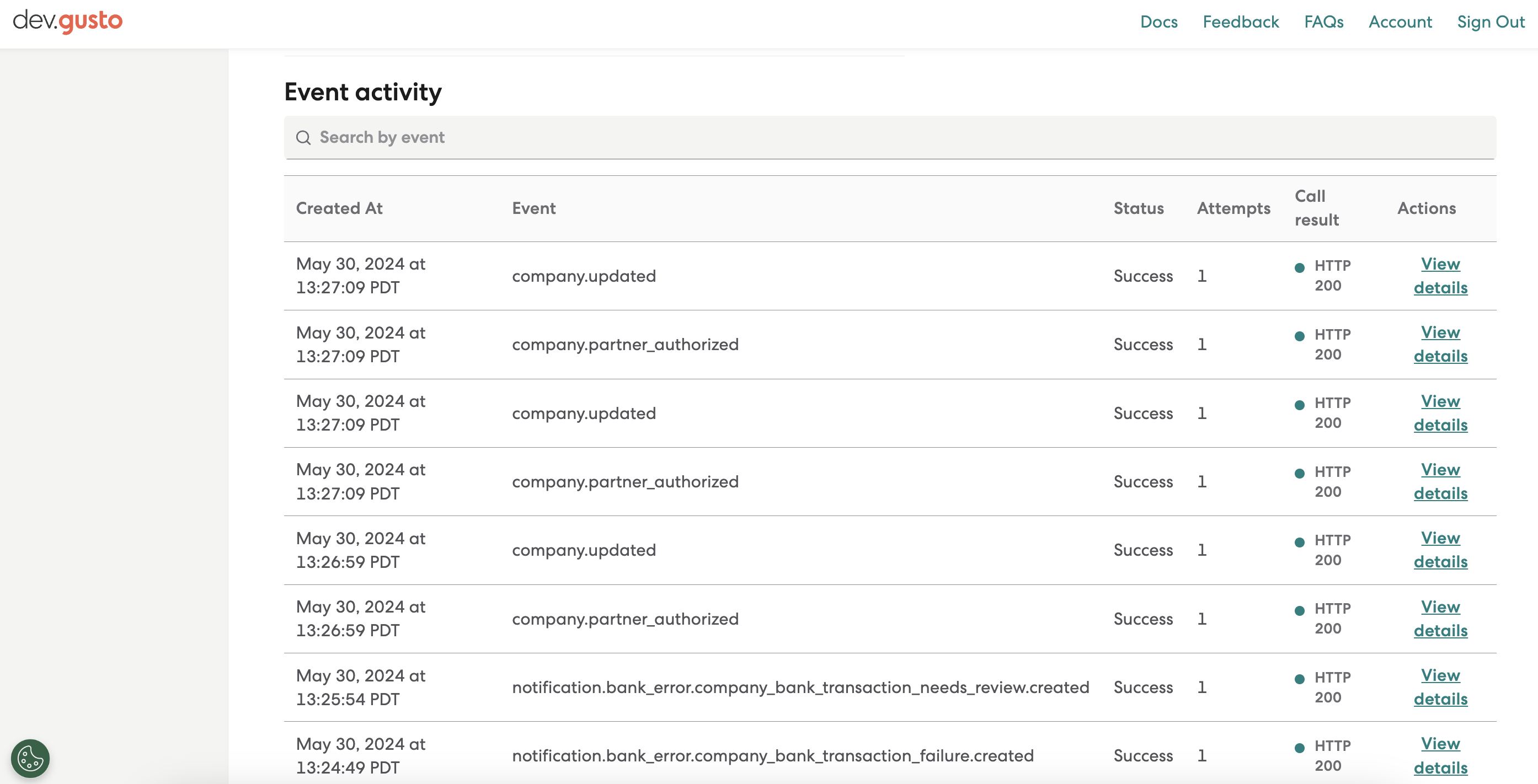
Setting up via the API
1. Set up an HTTPS endpoint in your application that can accept webhook requests via a POST method.
Set up an HTTPS endpoint in your application that can accept webhook requests via a POST method.
Your endpoint should be able to handle POST requests with a JSON payload, and should quickly return a 2xx successful response within 10 seconds.
In absence of this acknowledgement, our system will assume delivery failure and continue to retry up to 16 times over the course of 3 days using an exponential backoff scheme.
2. Create a webhook subscription
When you create a webhook subscription, you should provide an initial set of subscription types that you’d like your application to receive. You may update this subscription at a later time. See all webhook event types here.
The url
noted here should be your application’s webhook URL. Once created, the webhook subscription should be in pending
status.
curl --request POST \
--url https://api.gusto-demo.com/v1/webhook_subscriptions \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--header 'Authorization: Token API_TOKEN'\
--data '
{
"subscription_types": [
"Company",
"Employee"
],
"url": "https://leading-role-app.com/subscriber"
}
'
const fetch = require('node-fetch');
const url = 'https://api.gusto-demo.com/v1/webhook_subscriptions';
const options = {
method: 'POST',
headers: {accept: 'application/json', 'content-type': 'application/json'},
body: JSON.stringify({
subscription_types: ['Company', 'Employee'],
url: 'https://leading-role-app.com/subscriber'
})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
3. Verify your new webhook subscription
Once the subscription has been created, we will send a POST request to your webhooks URL with a verification_token
. This step helps Gusto validate that webhook events are reaching its intended destination, and helps you troubleshoot any issues with webhook delivery.
If your endpoint does not receive a verification_token
, please ensure that your webhooks endpoint is set up correctly and actively listening.
Once you receive a verification_token
, call the PUT webhook_subscriptions/{webhook_subscription_uuid}/verify endpoint to verify the endpoint and begin receiving webhook events.
We recommend that you save this verification_token
for the next step. This token is used to help you verify that events were sent by Gusto.
curl --request PUT \
--url https://api.gusto-demo.com/v1/webhook_subscriptions/{webhook_subscription_uuid}/verify \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"verification_token": "asefasedfe23e234easd"
}
'
const fetch = require('node-fetch');
const url = 'https://api.gusto-demo.com/v1/webhook_subscriptions/{webhook_subscription_uuid}/verify';
const options = {
method: 'PUT',
headers: {accept: 'application/json', 'content-type': 'application/json'},
body: JSON.stringify({verification_token: 'asefasedfe23e234easd'})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
Updated 8 months ago